Data Structure Designer | JavaScript
Alternative Programming Languages for Resolving Dependencies and
This analysis explores alternative programming languages like TypeScript, Python, and Ruby that can be used in conjunction with Express.js to resolve dependencies and add user authentication middleware. Example code in TypeScript demonstrates how
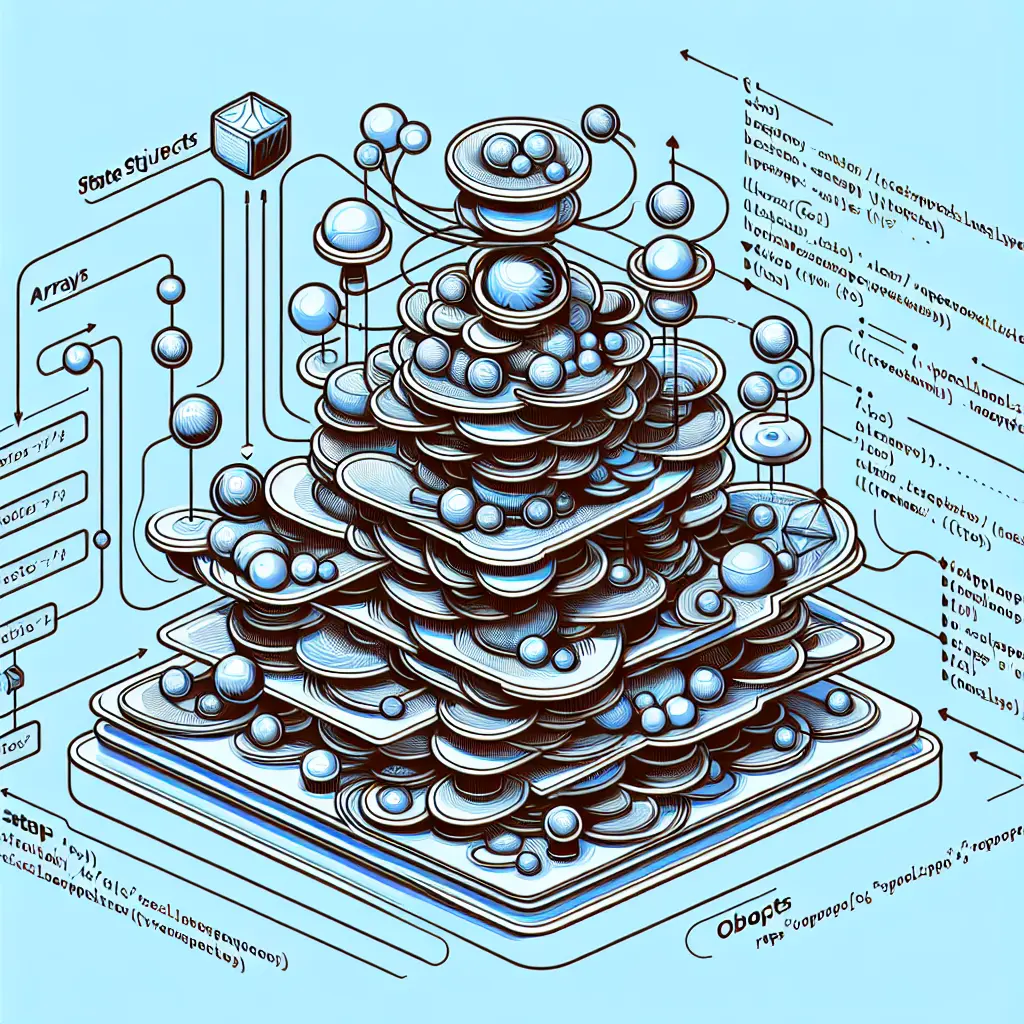
Prompt
Are there any alternative programming languages besides JavaScript that can be used to resolve dependencies and add user authentication middleware to an Express.js server? If yes, provide an example or explain how it can be achieved.
Answer
Problem Description
The problem is to find alternative programming languages other than JavaScript that can be used to resolve dependencies and add user authentication middleware to an Express.js server. We need to provide an example or explain how it can be achieved using these alternative programming languages.
Solution
There are several alternative programming languages that can be used to resolve dependencies and add user authentication middleware to an Express.js server. Some of these languages include:
TypeScript: TypeScript is a statically-typed superset of JavaScript that compiles to plain JavaScript. It provides features like type checking and compile-time error checking. To resolve dependencies in TypeScript, you can use a package manager like NPM or Yarn, and add user authentication middleware using libraries like Passport.
Python: Python is a popular programming language that can be integrated with Express.js using frameworks like Flask or Django. You can use Python package managers like pip or conda to install dependencies, and add user authentication middleware using libraries like Flask-Login or Django-AllAuth.
Ruby: Ruby is another language that can be used alongside Express.js by using frameworks like Ruby on Rails or Sinatra. You can use Ruby package managers like Bundler to handle dependencies, and add user authentication middleware using libraries like Devise or OmniAuth.
Here is a template in TypeScript for resolving dependencies and adding user authentication middleware to an Express.js server:
// Dependencies resolution
import express from 'express';
import { json } from 'body-parser';
import passport from 'passport';
import { Strategy as LocalStrategy } from 'passport-local';
import { Strategy as JWTStrategy, ExtractJwt } from 'passport-jwt';
const app = express();
app.use(json());
// User authentication middleware using Passport
app.use(passport.initialize());
// Local strategy for username/password authentication
passport.use(new LocalStrategy((username, password, done) => {
// Your authentication logic here
}));
// JWT strategy for token-based authentication
passport.use(new JWTStrategy({
jwtFromRequest: ExtractJwt.fromAuthHeaderAsBearerToken(),
secretOrKey: 'your-secret-key',
}, (payload, done) => {
// Your authentication logic here
}));
// Your Express.js routes and server setup here
// ...
app.listen(3000, () => {
console.log('Express server listening on port 3000');
});
Note that the above code is written in TypeScript, but the same concepts can be applied in other languages like Python and Ruby. The specific libraries and syntax may differ, but the overall approach remains the same.
Conclusion
To resolve dependencies and add user authentication middleware to an Express.js server, you can use alternative programming languages like TypeScript, Python, or Ruby. These languages offer robust frameworks and libraries that can integrate well with Express.js and provide the required functionality.
Description
This analysis explores alternative programming languages like TypeScript, Python, and Ruby that can be used in conjunction with Express.js to resolve dependencies and add user authentication middleware. Example code in TypeScript demonstrates how to achieve this functionality, showcasing the use of libraries like Passport for authentication. These alternative languages offer robust frameworks and libraries that seamlessly integrate with Express.js to provide the necessary features for managing dependencies and user authentication.