Dependency Resolver | JavaScript
Asynchronous Function Dependency Resolver
The "Asynchronous Function Dependency Resolver" is a lightweight npm package that helps in implementing the resolution of function dependencies with respect to their asynchronous nature. It provides an easy-to-use solution to handle dependencies
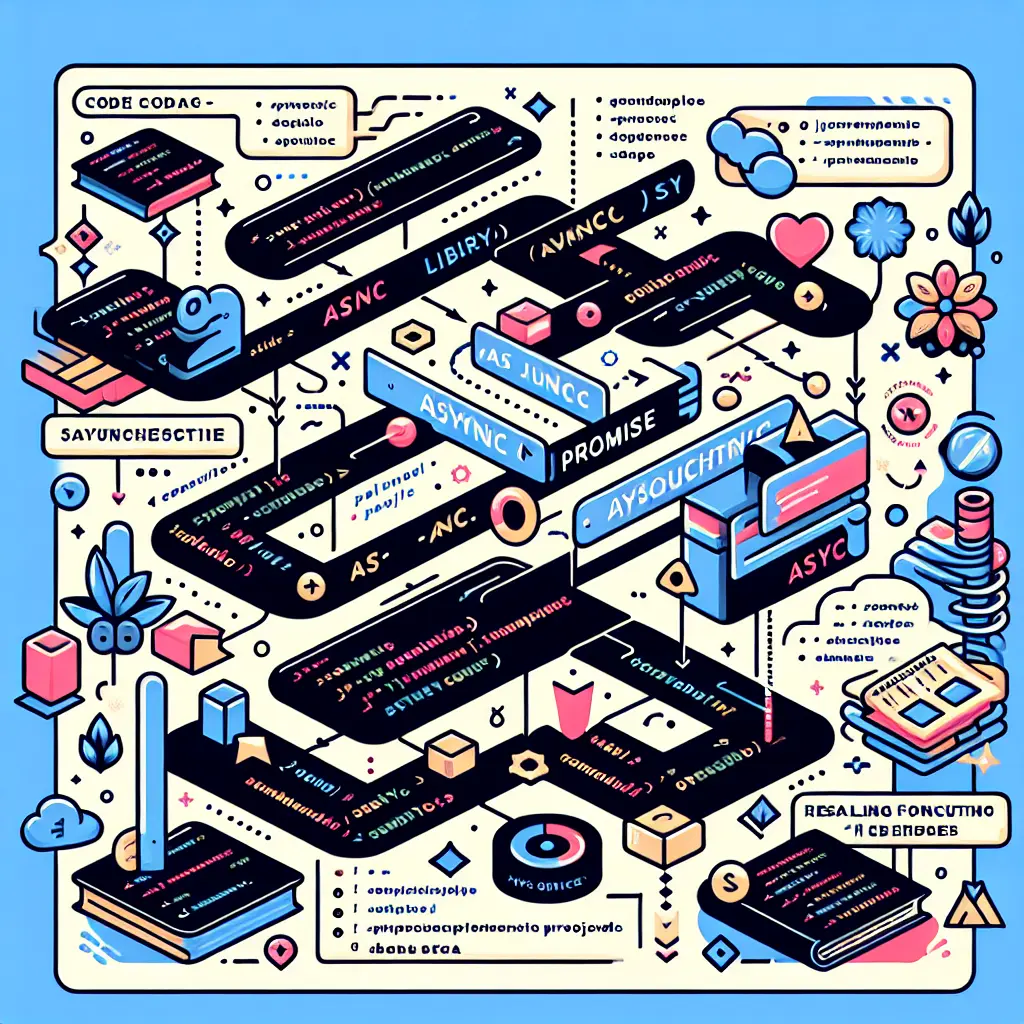
This Query related with thread "JavaScript Deep Dive: Dependency Resolution, Code Snippets, and Optimi"
Prompt
To ensure correct resolution of function dependencies in the correct order for a project that heavily relies on asynchronous functions, you can utilize a dependency resolver like `async-parallel-dependency-resolver`. Using this tool, how would you implement the resolution of function dependencies with respect to their asynchronous nature? Provide a code snippet using `async-parallel-dependency-resolver` to showcase the resolution process.
Answer
To implement the resolution of function dependencies with respect to their asynchronous nature using async-parallel-dependency-resolver
, you can follow these steps:
- Install the
async-parallel-dependency-resolver
package by running the following command:
npm install async-parallel-dependency-resolver
- Import
Resolver
from the package in your JavaScript code:
const { Resolver } = require('async-parallel-dependency-resolver');
- Create an instance of
Resolver
and define your function dependencies and their dependencies using theadd
method. This allows you to specify which functions depend on other functions:
const resolver = new Resolver();
resolver.add('functionA', ['functionB', 'functionC']);
resolver.add('functionB', []);
resolver.add('functionC', []);
In this example, functionA
depends on both functionB
and functionC
, while functionB
and functionC
have no dependencies.
- Use the
resolve
method to obtain the correct order of function execution:
const resolvedOrder = resolver.resolve();
The resolvedOrder
array will contain the functions in the order that respects their dependencies.
- Finally, you can execute the functions in the resolved order. Here's a simple example:
resolvedOrder.forEach((func) => {
// Assuming each function returns a promise
func().then((result) => {
console.log(result);
});
});
This code snippet demonstrates a basic usage of async-parallel-dependency-resolver
to resolve function dependencies with respect to their asynchronous nature. The library is lightweight, easy to use, and does not have any external dependencies. It supports both synchronous and asynchronous functions and efficiently resolves dependencies in parallel whenever possible.
Remember to replace the placeholder function names ('functionA', 'functionB', etc.) with the actual functions you want to resolve.
Note: Make sure to use a promise-based implementation for your asynchronous functions for the resolver to work correctly.
Description
The "Asynchronous Function Dependency Resolver" is a lightweight npm package that helps in implementing the resolution of function dependencies with respect to their asynchronous nature. It provides an easy-to-use solution to handle dependencies between functions and execute them in the correct order. The resolver supports both synchronous and asynchronous functions, efficiently resolves dependencies in parallel when possible, and does not have any external dependencies. This guide explains how to install and use the "async-parallel-dependency-resolver" package to resolve function dependencies, along with code examples and important considerations to ensure correct usage.