Design Pattern Implementer | Excel
Capitalizing Text in a Column using Strategy Design Pattern
This implementation demonstrates how to capitalize all the text in a column using the Strategy design pattern, which allows for encapsulating different strategies for capitalizing text. The code provides examples of implementing and using different s...
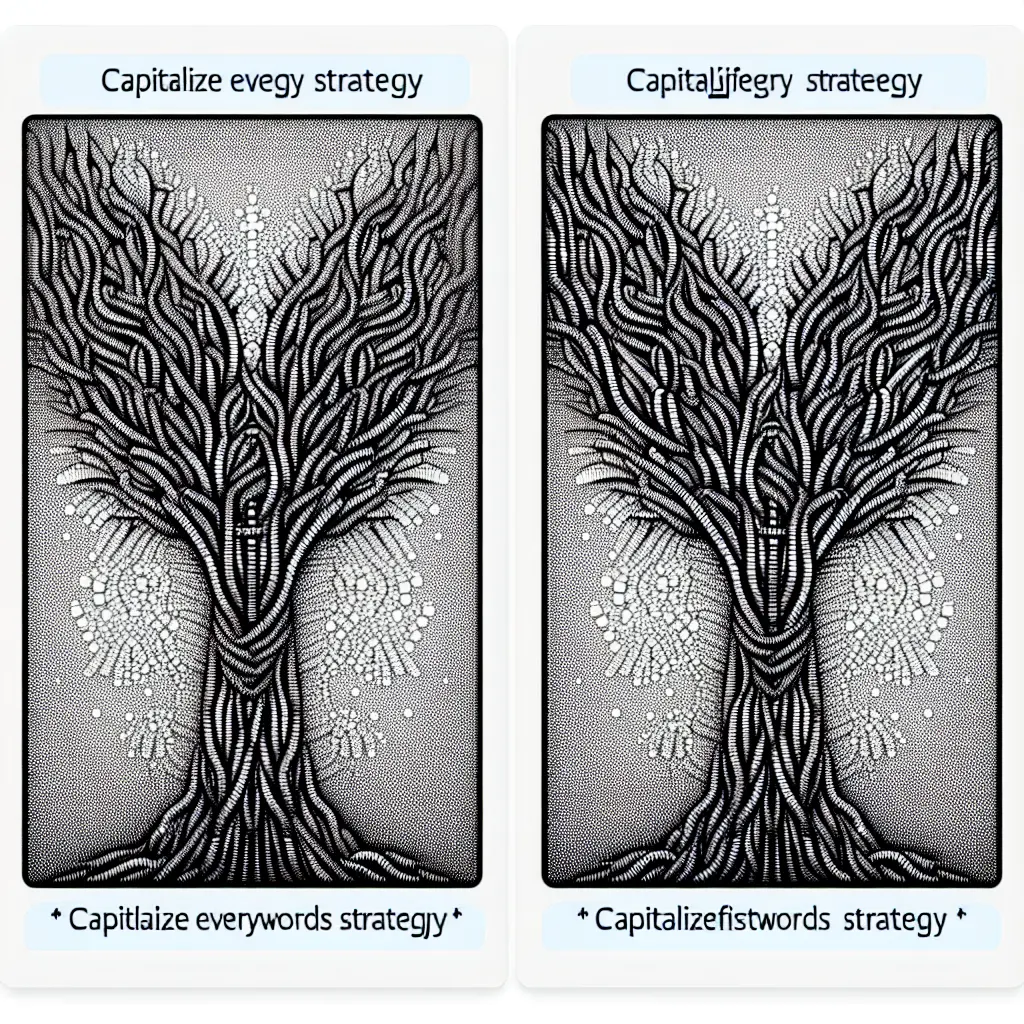
Prompt
How can I capitalize all the text in a column
Answer
To capitalize all the text in a column, we can use the Strategy design pattern.
Strategy Design Pattern
The Strategy design pattern allows us to encapsulate different algorithms and make them interchangeable. In this case, we can have different strategies for capitalizing text, such as capitalizing every word or capitalizing only the first letter of each word.
Implementation
Here's an example of how we can implement the Strategy design pattern to capitalize all the text in a column. Let's assume we have a DataFrame with a column called 'text' that we want to capitalize.
- Define an interface (
TextCapitalizer
) that declares a method for capitalizing text. - Implement different strategies that implement the
TextCapitalizer
interface, such asCapitalizeEveryWordStrategy
andCapitalizeFirstLetterStrategy
. - In your code, instantiate the desired strategy based on your requirements. For example, create an instance of
CapitalizeEveryWordStrategy
orCapitalizeFirstLetterStrategy
. - Use the instantiated strategy to capitalize the text in the column.
Below is a Python code snippet that demonstrates this implementation:
# Define the TextCapitalizer interface
class TextCapitalizer:
def capitalize(self, text):
pass
# Implement the CapitalizeEveryWordStrategy
class CapitalizeEveryWordStrategy(TextCapitalizer):
def capitalize(self, text):
return ' '.join([word.capitalize() for word in text.split()])
# Implement the CapitalizeFirstLetterStrategy
class CapitalizeFirstLetterStrategy(TextCapitalizer):
def capitalize(self, text):
return ' '.join([word[0].upper() + word[1:] for word in text.split()])
# Instantiate the desired strategy
cap_strategy = CapitalizeEveryWordStrategy()
# Capitalize the text in the column
df['text'] = df['text'].apply(lambda x: cap_strategy.capitalize(x))
This implementation allows us to easily switch between different capitalization strategies by instantiating the appropriate strategy object. Additionally, it separates the algorithm for capitalization from the code using it, promoting code reusability and maintainability.
Note: The code above assumes that you are already working with a DataFrame object, and the 'text' column exists. Please modify the code accordingly based on your specific requirements.
Description
The implementation focuses on capitalizing text in a column of a DataFrame using the Strategy design pattern. The Strategy pattern allows encapsulating different algorithms for capitalizing text and facilitates switching between strategies. The code defines an interface TextCapitalizer
that declares the capitalize
method for capitalizing text. It then provides two strategy implementations: CapitalizeEveryWordStrategy
and CapitalizeFirstLetterStrategy
, both implementing the TextCapitalizer
interface. By instantiating the desired strategy object (cap_strategy
), the code can be easily switched between different strategies. The df['text'].apply
method applies the chosen strategy to capitalize the text in the column. This implementation helps in improving code reusability and maintainability.