Code Generator | JavaScript
Creating Objects in JavaScript
This article explains different methods for creating objects in JavaScript. It covers the object literal syntax, object constructor, and ES6 class syntax. It also provides examples of how to access, modify, and delete object properties and methods.
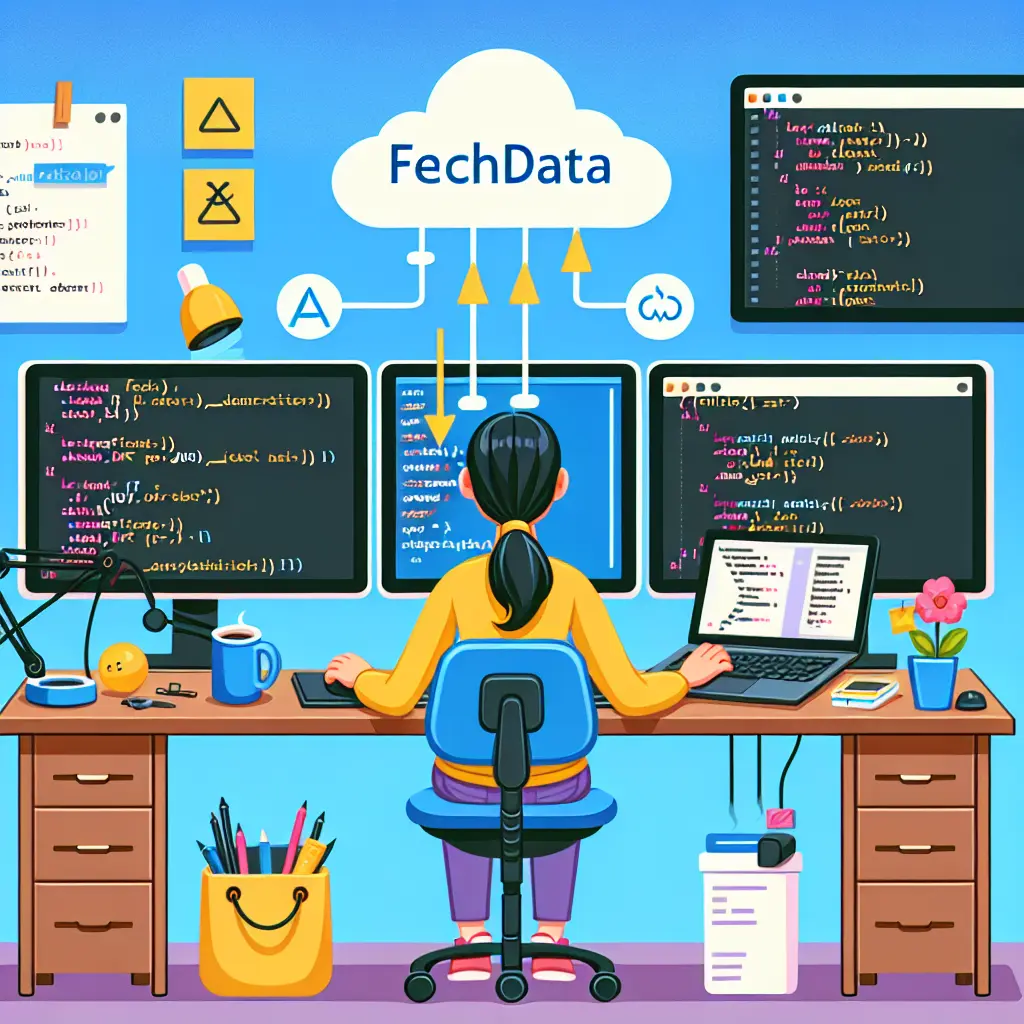
This Query related with thread "JavaScript Deep Dive: Dependency Resolution, Code Snippets, and Optimi"
Prompt
mace object
Answer
To make an object in JavaScript, you can use either the object literal syntax or the Object constructor. Here's an example of how you can create an object using both methods:
Using object literal syntax:
const obj1 = {
property1: 'value1',
property2: 'value2',
method1: function() {
// do something
}
};
Using the Object constructor:
const obj2 = new Object();
obj2.property1 = 'value1';
obj2.property2 = 'value2';
obj2.method1 = function() {
// do something
};
You can access the properties and methods of the object using dot notation or square bracket notation:
console.log(obj1.property1); // Output: 'value1'
console.log(obj2['property2']); // Output: 'value2'
obj1.method1(); // call the method
You can also modify and delete properties dynamically:
obj1.property1 = 'new value'; // modify a property
delete obj2.property2; // delete a property
It's also possible to create an object using ES6 class syntax:
class MyClass {
constructor(property1, property2) {
this.property1 = property1;
this.property2 = property2;
}
method1() {
// do something
}
}
const obj3 = new MyClass('value1', 'value2');
console.log(obj3.property1); // Output: 'value1'
obj3.method1(); // call the method
Remember to include proper error handling and validation, such as checking if the object constructor or properties exist before accessing or modifying them.
Description
This article explains different methods for creating objects in JavaScript. It covers the object literal syntax, object constructor, and ES6 class syntax. It also provides examples of how to access, modify, and delete object properties and methods. Additionally, it emphasizes the importance of proper error handling and validation.