Code Explainer | SQL
CURSOR Function for Iterating Through Result Sets
This code snippet demonstrates the usage of the `CURSOR` function to define and initialize two cursors, `cursor1` and `cursor2`. These cursors are used to retrieve and process data from `table1` and `table2` respectively. The code shows the declarati...
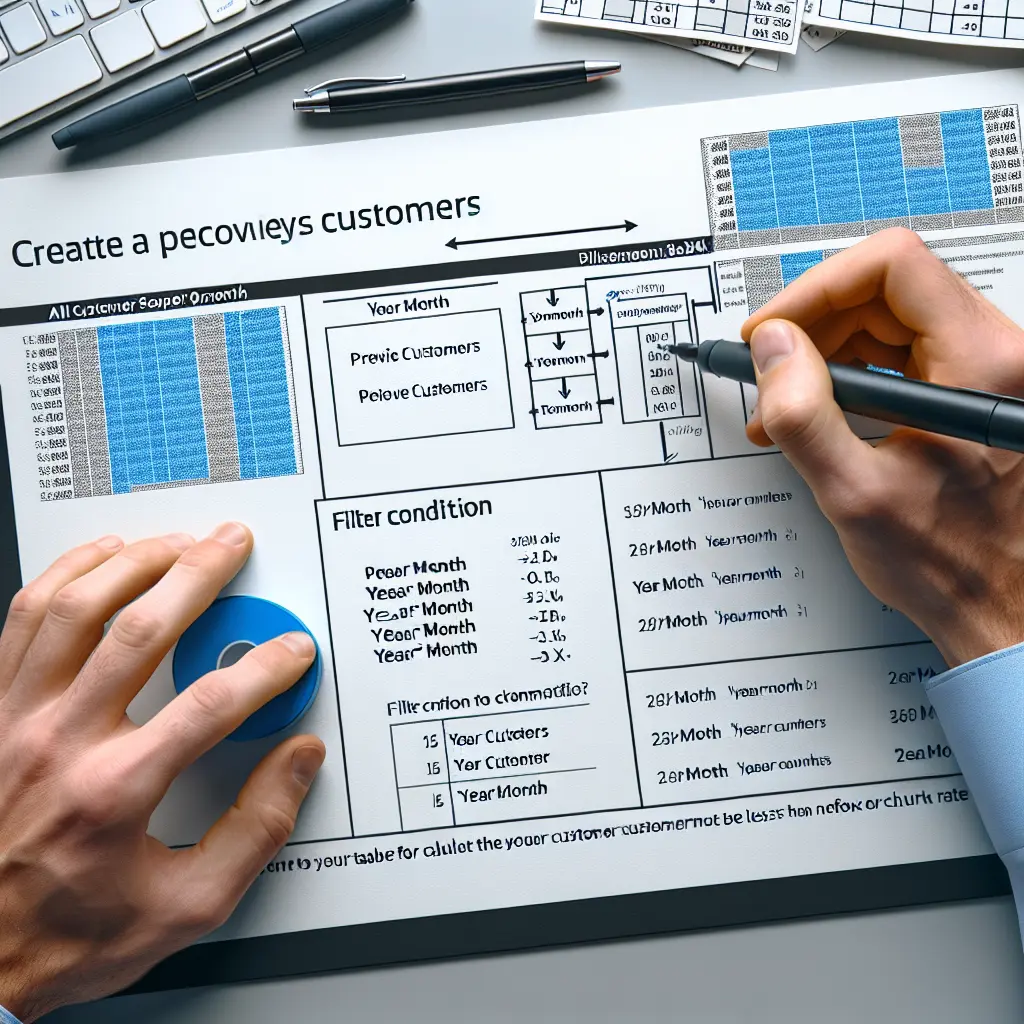
This Query related with thread "SQL Function with Multiple Loops"
Prompt
DECLARE
cursor1 CURSOR FOR SELECT column1 FROM table1; -- Define the cursor
cursor2 CURSOR FOR SELECT column2 FROM table2; -- Define another cursor
variable1 INT; -- Variable for cursor1
variable2 INT; -- Variable for cursor2
what is the CURSOR function doing here
Answer
The CURSOR
function in the given code is used to define and initialize two cursors: cursor1
and cursor2
.
cursor1
: This cursor is defined to select values fromcolumn1
intable1
.cursor2
: This cursor is defined to select values fromcolumn2
intable2
.
Both cursors will be used to retrieve and process data from their respective tables.
Using cursors allows you to iterate through the result set of a query one row at a time. Cursors are often used in database programming to perform operations on each row of a result set individually.
Variables variable1
and variable2
are also declared and associated with cursor1
and cursor2
respectively. These variables will be used to store the values retrieved by the cursors.
It's important to note that the given code snippet does not provide information about how the cursors and variables are being used further in the code. The usage of cursors typically involves operations like fetching data, updating values, or performing calculations.
Example:
DECLARE
cursor1 CURSOR FOR SELECT employee_name FROM employees_table;
emp_name VARCHAR2(50);
BEGIN
OPEN cursor1;
LOOP
FETCH cursor1 INTO emp_name;
EXIT WHEN cursor1%NOTFOUND;
-- Perform operations on emp_name
-- e.g. PRINT emp_name;
END LOOP;
CLOSE cursor1;
END;
In the example above, a cursor named cursor1
is defined to select employee_name
from the employees_table
. The cursor is then opened and a loop is used to fetch each row's employee_name
into the variable emp_name
. Operations can be performed on emp_name
within the loop. Finally, the cursor is closed to release resources.