Design Pattern Implementer | Excel
Decorator Pattern for Capital Letters
The Decorator Pattern allows us to dynamically add new functionality to a column of data without modifying its original implementation. In this case, we can use the Decorator Pattern to convert the data in a column to capital letters. This makes the...
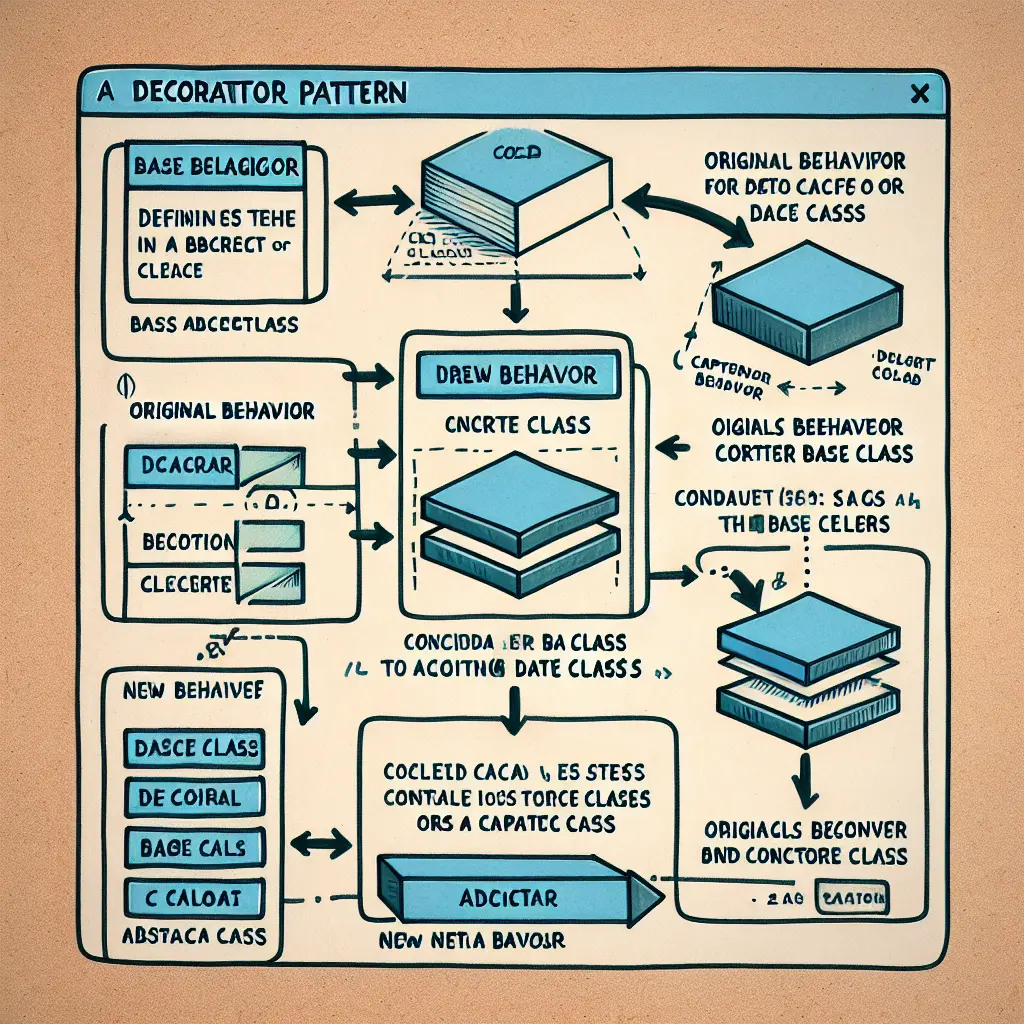
Prompt
How can I create capital letters for a column of data
Answer
Design Pattern: Decorator Pattern
The Decorator Pattern allows us to dynamically add new functionality to an object without modifying its structure. In the context of creating capital letters for a column of data, we can use the Decorator Pattern to enhance the existing functionality of the column and convert the data to capital letters.
Implementation Steps:
- Create an interface or abstract class that defines the base behavior for the column.
- Implement a concrete class that represents the original behavior of the column.
- Create a decorator class that extends the base class/interface and wraps an instance of the base class.
- Add a new behavior to the decorator class that converts the data to capital letters.
- Use the decorator class to enhance the behavior of the original column.
Here's an example implementation in Python:
# Step 1: Define the base behavior for the column
class DataColumn:
def __init__(self, data):
self.data = data
def get_data(self):
return self.data
# Step 2: Implement the original behavior of the column
class OriginalColumn(DataColumn):
def __init__(self, data):
super().__init__(data)
# Step 3: Create the decorator class
class CapitalLettersColumn(DataColumn):
def __init__(self, original):
self.original = original
# Step 4: Add new behavior to the decorator class
def get_data(self):
data = self.original.get_data()
return data.upper()
# Step 5: Use the decorator class to enhance the behavior of the original column
data_column = OriginalColumn("example data")
decorated_column = CapitalLettersColumn(data_column)
capital_data = decorated_column.get_data() # Returns "EXAMPLE DATA"
In this implementation, the DataColumn
is the base behavior for the column, the OriginalColumn
is the concrete class that represents the original behavior, and the CapitalLettersColumn
is the decorator class that adds the new behavior of converting the data to capital letters. We create an instance of OriginalColumn
and then wrap it with an instance of CapitalLettersColumn
to enhance its behavior.
By following the Decorator Pattern, we can easily add new functionality to the column without modifying its original implementation. This makes the code more flexible and maintainable.
Description
The Decorator Pattern is a design pattern that allows us to add additional functionalities to an object dynamically without modifying its original implementation. In the context of converting a column of data to capital letters, we can use the Decorator Pattern to enhance the existing behavior of the column.
The implementation steps for the Decorator Pattern are as follows:
- Define the base behavior for the column using an interface or abstract class.
- Implement the original behavior of the column in a concrete class.
- Create a decorator class that extends the base class/interface and wraps an instance of the base class.
- Add the new behavior to the decorator class.
- Use the decorator class to enhance the behavior of the original column.
The provided example demonstrates the implementation of the Decorator Pattern in Python. The DataColumn
class serves as the base behavior for the column, the OriginalColumn
class represents the original behavior, and the CapitalLettersColumn
class is the decorator that converts the data to capital letters. By wrapping an instance of OriginalColumn
with CapitalLettersColumn
, we can easily add the functionality of converting the data to capital letters without modifying the existing code.
By utilizing the Decorator Pattern, we achieve a flexible and maintainable solution that allows us to enhance the behavior of the column without directly modifying its original implementation.