Code Explainer | Python
Generating Public URL for Cloud-Hosted File in Laravel
Learn how to use `Storage::cloud()->url($thumbnailUrl)` in Laravel to generate a publicly accessible URL for files stored in cloud services like Amazon S3 or Google Cloud Storage. Understand the key concepts, code breakdown, example
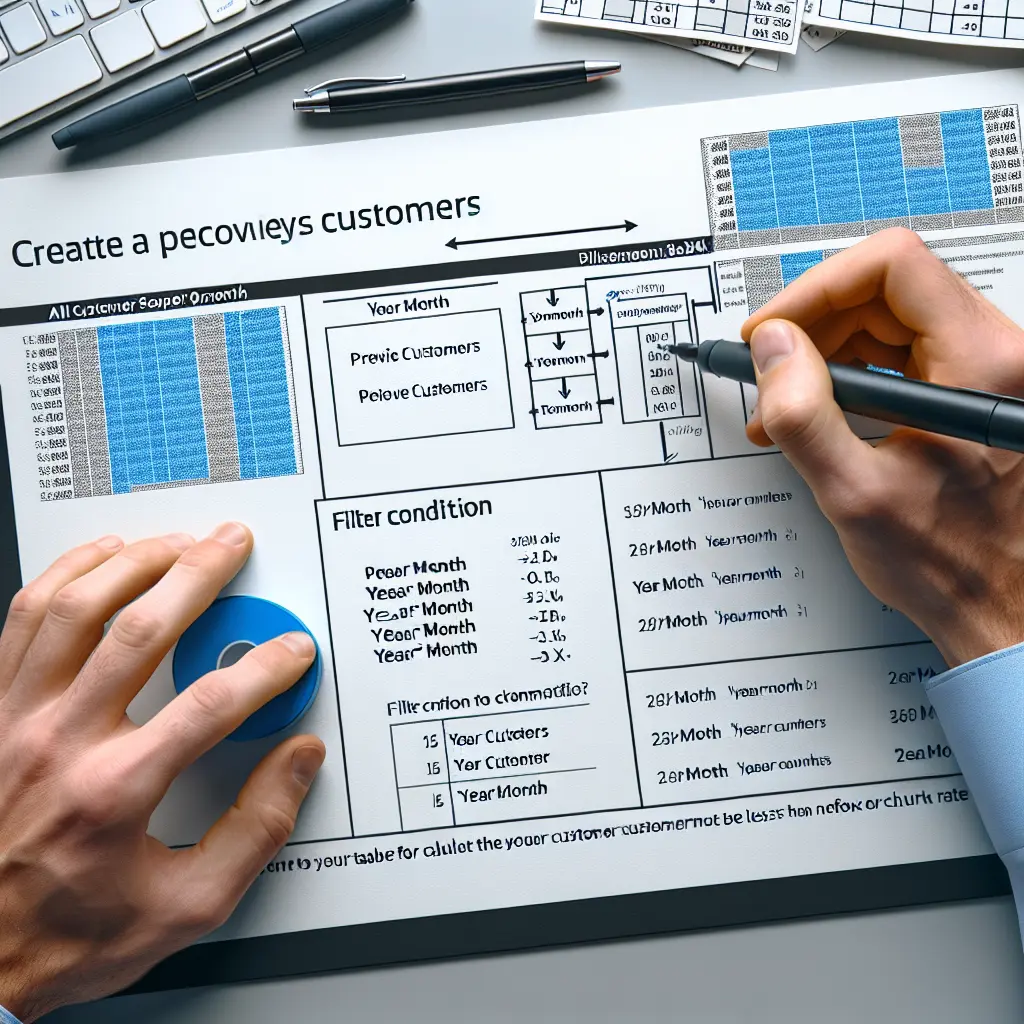
Prompt
Storage::cloud()->url($thumbnailUrl)
Answer
Explanation of Storage::cloud()->url($thumbnailUrl)
The code snippet Storage::cloud()->url($thumbnailUrl)
is written in PHP and is typically used in the context of Laravel, a popular PHP web framework. This code relates to file storage and retrieval.
Key Concepts
Storage:
- This is a facade provided by Laravel. A facade in Laravel provides a static-like interface to services within the application's service container.
- The
Storage
facade is used to interact with various file storage systems such as local disk, S3, and other cloud-based storage services.
cloud():
- The
cloud()
method is a shortcut to access the default cloud disk configured in theconfig/filesystems.php
file. - This configuration typically includes services like Amazon S3, Google Cloud Storage, or others.
- The
url($thumbnailUrl):
- This method generates a publicly accessible URL for a file stored on the cloud disk.
- The
$thumbnailUrl
variable should contain the path to the thumbnail image you want to generate a URL for.
Breaking Down the Code
Storage::cloud()->url($thumbnailUrl)
Storage::cloud()
:- This part of the code is telling Laravel to use the cloud storage disk defined in the application configuration.
- It ensures that the operations we are performing (in this case, generating a URL) are using the cloud storage as defined (e.g., S3, Google Cloud Storage).
->url($thumbnailUrl)
:- This function call generates a URL for the given file path (
$thumbnailUrl
). - The
$thumbnailUrl
should be a valid path to a file that's stored in the cloud storage.
- This function call generates a URL for the given file path (
Example Usage
Here is a practical example to illustrate the concept:
Laravel Configuration for Cloud Storage
- Ensure your
config/filesystems.php
is set up:
'cloud' => 's3', // Choosing Amazon S3 as the cloud disk
- Ensure your
Controller Example
// Assuming we have a thumbnail image stored in the S3 bucket $thumbnailPath = 'thumbnails/example.jpg'; // Generate a publicly accessible URL for the image $publicUrl = Storage::cloud()->url($thumbnailPath); // $publicUrl now holds the URL to access 'example.jpg'
Alternative Example
To further illustrate, here's an example of how to achieve the same with a local disk:
Local Disk Configuration
- Ensure your
config/filesystems.php
is set up:
'disks' => [ 'local' => [ 'driver' => 'local', 'root' => storage_path('app'), ], ],
- Ensure your
Controller Example
// Path to the file within the local storage $thumbnailPath = 'thumbnails/example.jpg'; // Generate a URL $publicUrl = Storage::disk('local')->url($thumbnailPath); // $publicUrl now holds the URL to access 'example.jpg' stored locally
Summary
- Purpose:
Storage::cloud()->url($thumbnailUrl)
is used to generate a publicly accessible URL for a file stored in the cloud. - Components: The
Storage
facade,cloud()
method, andurl()
function. - Usage: Commonly used for accessing images, documents, or other files stored remotely on cloud services.
For those looking to deepen their understanding, consider exploring relevant courses on the Enterprise DNA Platform, especially those focusing on web development and cloud services integration.
Description
Learn how to use Storage::cloud()->url($thumbnailUrl)
in Laravel to generate a publicly accessible URL for files stored in cloud services like Amazon S3 or Google Cloud Storage. Understand the key concepts, code breakdown, example usage, and its significance in web development.