Documentation Generator | SQL
Input Validation and Sanitization in Dynamic SQL Queries
This code documentation showcases how to ensure input validation and sanitization when executing dynamic SQL queries. It explains the use of parameterized queries to mitigate SQL injection risks and how to handle errors using a TRY-CATCH block. Testi...
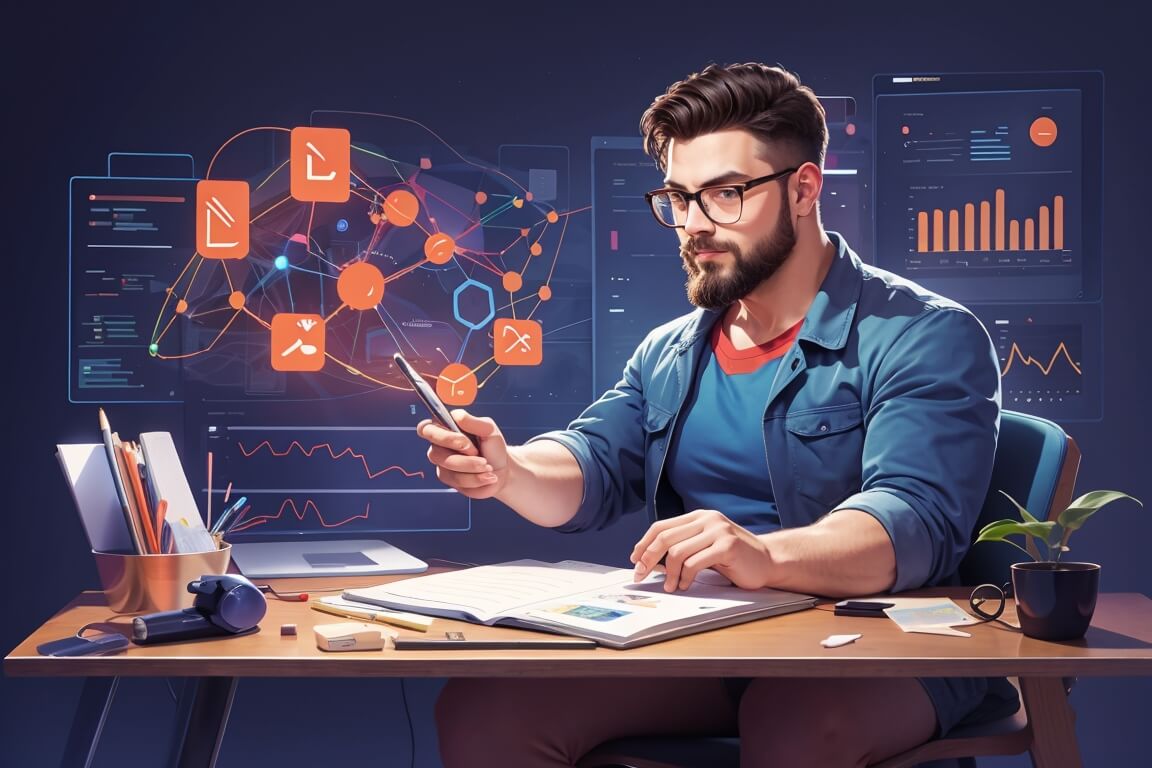
This Query related with thread "Guidelines for Working with Dynamic SQL Queries"
Prompt
-- Ensure input validation and sanitization
DECLARE @inputValue NVARCHAR(50);
SET @inputValue = 'Robert''; DROP TABLE Student;--';
-- Use parameterized query
DECLARE @sqlQuery NVARCHAR(MAX);
SET @sqlQuery = N'SELECT * FROM Students WHERE Name = @name';
EXEC sp_executesql @sqlQuery, N'@name NVARCHAR(50)', @name = @inputValue;
-- Error handling
BEGIN TRY
-- Execute dynamic SQL
EXEC sp_executesql @sqlQuery, N'@name NVARCHAR(50)', @name = @inputValue;
END TRY
BEGIN CATCH
-- Log and handle the error
SELECT ERROR_NUMBER(), ERROR_MESSAGE(), GETDATE();
END CATCH
-- Testing and validation
DECLARE @parameterValue NVARCHAR(50);
SET @parameterValue = 'John';
SET @sqlQuery = N'SELECT * FROM Students WHERE Name = @name';
EXEC sp_executesql @sqlQuery, N'@name NVARCHAR(50)', @name = @parameterValue;
-- Perform testing with different input values and edge cases
Answer
Code Documentation - Ensure Input Validation and Sanitization
This code snippet showcases how to ensure input validation and sanitization when executing dynamic SQL queries.
Input Validation
- The variable
@inputValue
is used to store the input value. In this case, it is set to'Robert''; DROP TABLE Student;--'
to demonstrate a potential SQL injection vulnerability.
Sanitization and Parameterized Query
- To mitigate SQL injection risks, a parameterized query is used.
- The variable
@sqlQuery
is declared to store the SQL query with a placeholder@name
parameter. - The
sp_executesql
system stored procedure is then executed with the query and the@name
parameter definition.- The
@name
parameter is set to the@inputValue
value. - This ensures that the input value is treated as data and not executable code.
- The
Error Handling
- To handle errors, the dynamic SQL execution is wrapped inside a
TRY-CATCH
block. - If an error occurs during execution, it is caught in the
CATCH
block.- The error details are retrieved using
ERROR_NUMBER()
,ERROR_MESSAGE()
, andGETDATE()
. - These details can be logged or used for further error handling.
- The error details are retrieved using
Testing and Validation
- To test with different input values and edge cases, a new input value is assigned to
@parameterValue
.- In this example, it is set to
'John'
.
- In this example, it is set to
- The
sp_executesql
is then executed again with the new input value to validate the functionality.
It is important to note that this code snippet focuses on input validation and sanitization techniques within the context of dynamic SQL execution. Additional security measures, such as server-side input validation, should be implemented to strengthen the overall security of the application.
Description
This code documentation provides a comprehensive guide on how to ensure input validation and sanitization when executing dynamic SQL queries. It highlights the potential risks of SQL injection and demonstrates the use of parameterized queries to prevent them. The code also includes error handling using a TRY-CATCH block to capture and handle any exceptions that may occur during execution. Additionally, testing and validation steps are provided to demonstrate the functionality of the code with different input values. It emphasizes the importance of implementing server-side input validation to further enhance the security of the application.