Performance Predictor | JavaScript
JavaScript Code Optimization Overview
This analysis focuses on optimizing a given JavaScript code snippet that creates and appends a new paragraph element to an HTML element. The main points emphasize the performance bottleneck caused by repeated DOM accesses and propose alternative
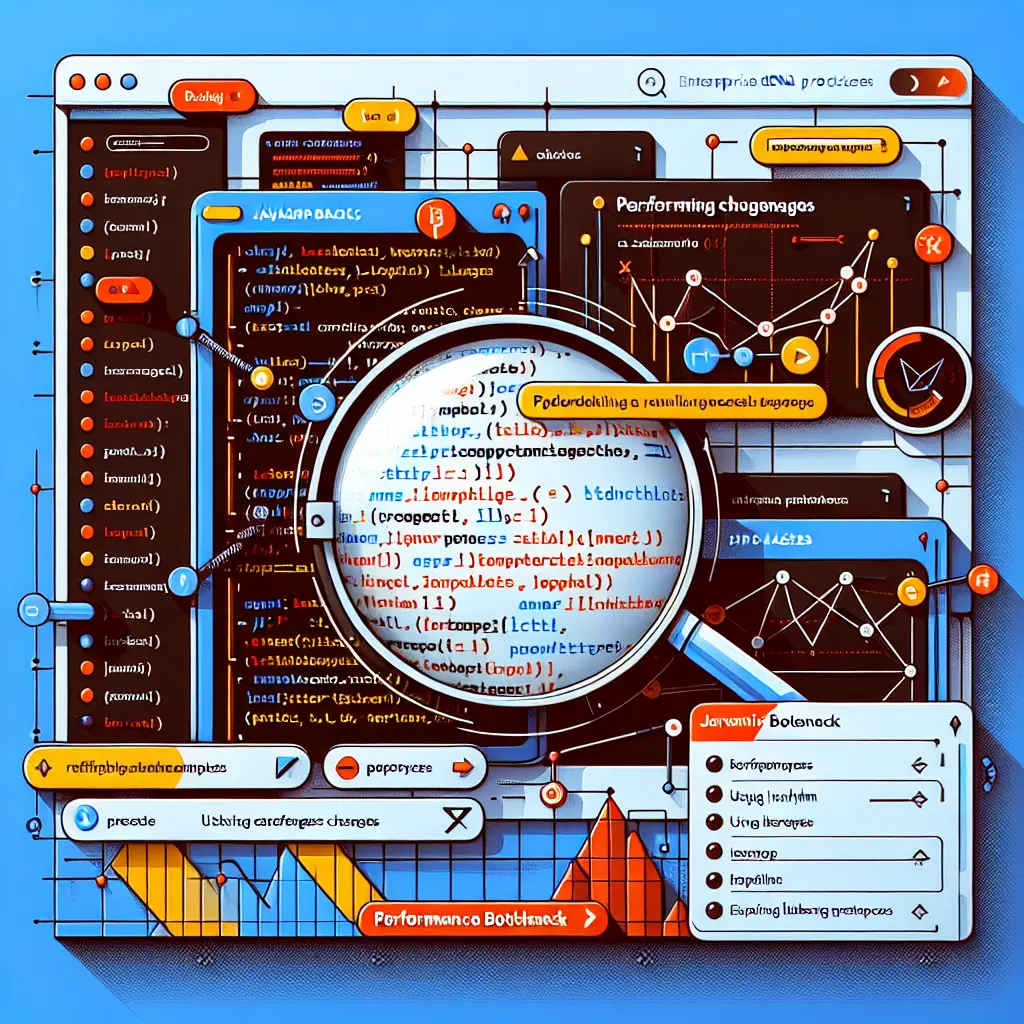
This Query related with thread "JavaScript Deep Dive: Dependency Resolution, Code Snippets, and Optimi"
Prompt
// Select an HTML element by its ID
var outputElement = document.getElementById("output");
// Create a new paragraph element
var paragraph = document.createElement("p");
// Set the text content of the paragraph
paragraph.textContent = "Hello, World!";
// Append the paragraph to the selected element
outputElement.appendChild(paragraph);
Answer
Language: JavaScript
Main Points:
- The given code snippet performs a simple operation of creating a new paragraph element and appending it to an HTML element.
- The performance bottleneck in this code snippet is the repeated access to the DOM, which can be expensive and slow down the code execution.
- The code snippet could be optimized by reducing the number of DOM accesses and batching multiple changes together.
- The alternative approach involves creating the entire paragraph element with its content in one go and then appending it to the output element, reducing the number of individual DOM operations.
Recommendations:
- Reduce DOM access: Instead of accessing the output element by its ID multiple times, it can be stored in a variable for reuse.
- Batch changes: Combine all the operations on the paragraph element before appending it to the output element. This reduces the number of individual DOM operations.
- Use innerHTML: Instead of setting the text content using
textContent
, consider usinginnerHTML
to set the entire HTML content of the paragraph, if applicable. However, make sure to sanitize any user-generated or untrusted data to prevent XSS attacks. - Consider using a library: If this operation is repeated frequently or if there are more complex operations involved, consider using a library like jQuery or React, which optimize DOM operations and provide additional performance benefits.
By following these recommendations, the code snippet can be optimized for better performance and efficiency. If you are interested in learning more about JavaScript coding practices, you can explore the "JavaScript Basics" and "JavaScript Best Practices" courses available on the Enterprise DNA platform.
Description
This analysis focuses on optimizing a given JavaScript code snippet that creates and appends a new paragraph element to an HTML element. The main points emphasize the performance bottleneck caused by repeated DOM accesses and propose alternative approaches to reduce the number of operations. Recommendations include reducing DOM access, batching changes, considering the use of innerHTML
, and exploring libraries like jQuery or React for more complex operations. Implementing these recommendations will enhance the code's performance and efficiency. Additional learning resources on JavaScript coding practices, including "JavaScript Basics" and "JavaScript Best Practices," are available on the Enterprise DNA platform.