Performance Predictor | SQL
Optimizing SQL Function with Multiple Loops
This code analysis provides recommendations for optimizing a SQL function that utilizes multiple loops. The recommendations include minimizing query calls, performing bulk operations, optimizing query performance, utilizing set-based operations, avoi...
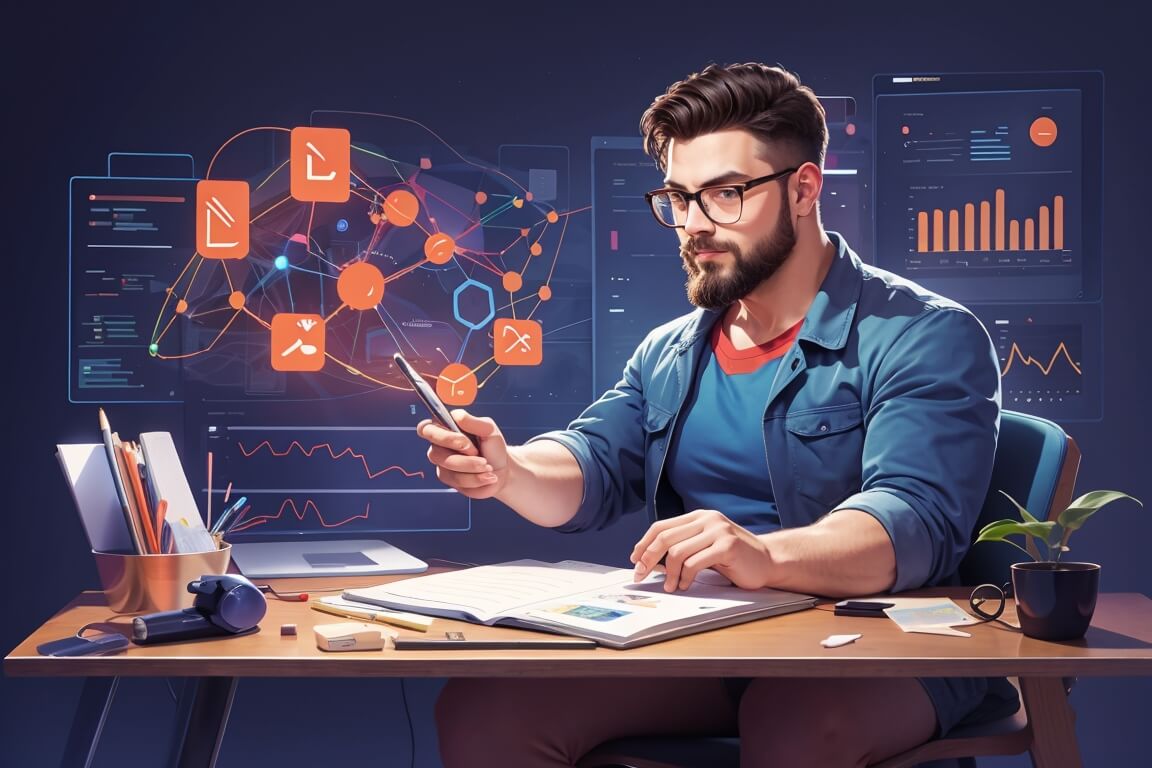
This Query related with thread "SQL Function with Multiple Loops"
Prompt
How can you optimize the performance of the SQL function with multiple loops? Provide specific techniques or SQL features that can be used.
Answer
Code Analysis:
The SQL function with multiple loops can introduce performance bottlenecks due to the iterative nature of the loops. Each iteration can result in additional database queries or operations, which can be expensive and impact performance.
Recommendations:
Minimize Query Calls: Instead of making queries within the loop, try to combine the logic and use SQL features like joins, subqueries, or conditional expressions to reduce the number of queries. This will minimize the overhead of query execution and network latency.
Bulk Operations: If possible, perform bulk operations or batch processing instead of processing each record individually within the loop. This can be achieved by using features like the INSERT INTO SELECT statement or the UPDATE statement with JOIN to update multiple records at once.
Optimize Query Performance: Ensure that your queries are optimized by having appropriate indexes, using the correct join types, and avoiding unnecessary sorting or filtering. Analyze the query execution plan to identify any potential optimizations.
Use Set-based Operations: Instead of processing data row by row, try to utilize set-based operations. This can be achieved by leveraging SQL features like aggregate functions or window functions, which can help perform computations on a set of data rather than row by row.
Avoid Nested Loops: If your function has nested loops, consider restructuring the logic to eliminate or reduce the nested loops. Nested loops can have a significant impact on performance, especially if the number of iterations is large.
Caching: If the data within the loops is not frequently changing, consider caching the data in memory using techniques like materialized views or temporary tables. This can reduce the need for repeated queries and improve performance.
Example:
Here's an example of optimizing a function with multiple loops using some of the recommendations mentioned above:
-- Original code with multiple loops
DECLARE @Result TABLE (Col1 INT, Col2 INT);
DECLARE @Loop1Var INT, @Loop2Var INT;
SET @Loop1Var = 1;
WHILE @Loop1Var <= 10
BEGIN
SET @Loop2Var = 1;
WHILE @Loop2Var <= 10
BEGIN
INSERT INTO @Result (Col1, Col2)
VALUES (@Loop1Var, @Loop2Var);
SET @Loop2Var = @Loop2Var + 1;
END
SET @Loop1Var = @Loop1Var + 1;
END
SELECT * FROM @Result;
Optimized code using set-based operations:
-- Optimized code using set-based operations
WITH CTE AS (
SELECT DISTINCT Number
FROM master.dbo.spt_values
WHERE Number BETWEEN 1 AND 10
)
INSERT INTO @Result (Col1, Col2)
SELECT C1.Number, C2.Number
FROM CTE C1
CROSS JOIN CTE C2;
SELECT * FROM @Result;
In this optimized code, we utilized a common table expression (CTE) to generate a set of numbers from 1 to 10. Then, we performed a cross join on the CTE to generate all the possible combinations of numbers from 1 to 10. Finally, we inserted the results into the @Result
table in a single statement, avoiding the need for loops and multiple queries. This set-based approach is more efficient and can significantly improve performance.