Performance Predictor
Performance Optimization for Parallel Encryption
This analysis highlights potential performance bottlenecks in a parallel encryption implementation and provides recommendations for optimization. It suggests incorporating dynamic chunking, asynchronous processing, measuring sequential encryption tim...
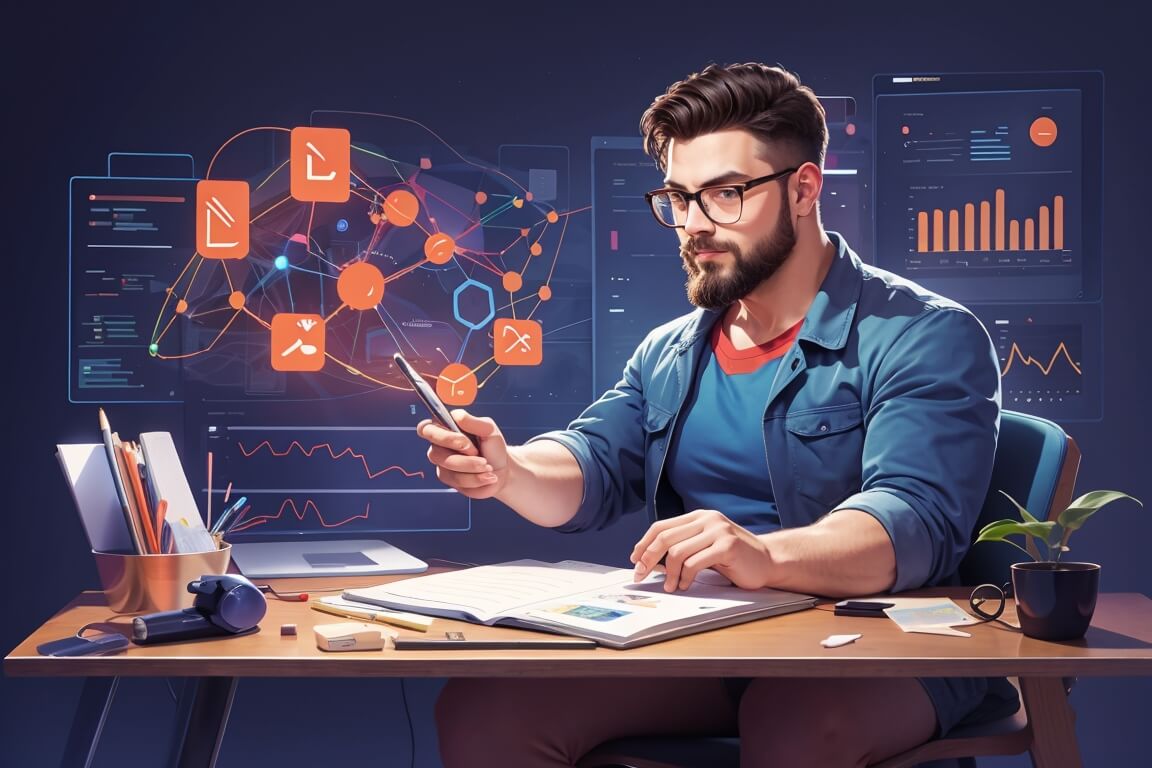
This Query related with thread "Code Generator AI for Python"
Prompt
# In order to delve deeper into performance impact analysis, let's take the concept of parallelization.
# Let's analyze its performance impact by implementing a simple parallel encryption function using the multiprocessing library in Python.
# The function should take a data string, divide it into chunks, and encrypt each chunk in a different processor core. Measure the time it takes for the function to complete.
# Make sure to also create a function that does the encryption sequentially for comparison purposes.
import time
import multiprocessing
from Crypto.Cipher import AES
key = b'Sixteen byte key'
aes = AES.new(key, AES.MODE_ECB)
# Define sequential and parallel encryption functions here
def encrypt_seq(data):
encrypted = aes.encrypt(data)
return encrypted
def encrypt_parallel(data_chunk):
encrypted = aes.encrypt(data_chunk)
return encrypted
# Define a function to split data into chunks
def split_data(data, num_cores):
chunk_size = len(data) // num_cores
chunks = [data[i:i+chunk_size] for i in range(0, len(data), chunk_size)]
return chunks
# Define the main function to call both parallel and sequential functions and measure time{0-#}
def main():
num_cores = multiprocessing.cpu_count()
data = 'Your data string here'.ljust(1024, '0')
chunks = split_data(data, num_cores)
# Spin up a pool of workers and apply encrypt_parallel function to each data chunk
pool = multiprocessing.Pool(processes=num_cores)
start = time.time()
pool.map(encrypt_parallel, chunks)
print('Parallel encryption time: ', time.time() - start)
# Now, do the encryption sequentially and measure time
start = time.time()
encrypt_seq(data)
print('Sequential encryption time: ', time.time() - start)
main()
Answer
Potential Performance Bottlenecks / Areas of Concern:
Data Chunking: The current implementation divides the input data into chunks based on the number of processor cores. However, the chunk size is predetermined based on the length of the data string. This approach may not lead to optimal performance. The chunk size should ideally be determined dynamically based on the available CPU resources and the size of the input data.
Parallel Function Execution: The
pool.map()
function is used to apply theencrypt_parallel
function to each data chunk in parallel. However, themap()
function has some performance overhead due to the need to divide the data and distribute it among the worker processes. This overhead can be significant for small chunks of data or when the encryption operation is relatively fast.Sequential Encryption: The sequential encryption function (
encrypt_seq
) is called after the parallel encryption is complete. However, the time taken by the sequential encryption is not accounted for in the final time calculation. This could lead to incorrect performance measurements.Task Distribution: The current implementation assigns each data chunk to a separate worker process using the
map()
function. However, depending on the size and complexity of the encryption operation, the workload of each chunk may not be evenly distributed. This can lead to some worker processes finishing their tasks earlier than others, causing potential idle time for those processes.
Recommendations for Optimization / Alternative Approaches:
Dynamic Chunking: Instead of using a fixed chunk size, it would be beneficial to have a more dynamic approach to determine the chunk size. This can be achieved by considering the available CPU resources (number of cores) and the size of the input data. For optimal performance, the chunk size should be balanced in a way that minimizes data distribution overhead while maximizing the utilization of CPU resources.
Asynchronous Processing: Instead of using
pool.map()
, which has performance overhead, an alternative approach would be to usepool.apply_async()
to submit each encryption task asynchronously. This will allow the main process to continue without waiting for the completion of each task, reducing the overall time taken.Measure Sequential Encryption Time: To ensure accurate performance measurements, the time taken by the sequential encryption function (
encrypt_seq
) should be measured and accounted for in the final time calculation. This can be done by capturing the start time before callingencrypt_seq
and subtracting it from the end time after the function call.Load Balancing: To ensure efficient utilization of CPU resources and minimize idle time, load balancing techniques can be employed. This can involve dynamically distributing the workload among worker processes based on factors such as execution time, CPU utilization, and the complexity of the encryption operation.
Overall, by implementing dynamic chunking, using asynchronous processing, measuring sequential encryption time, and incorporating load balancing techniques, the performance of the parallel encryption function can be significantly improved. Additionally, it is important to consider the specific requirements and characteristics of the encryption operation to optimize the code further.
Description
The input provided by the user discusses potential performance bottlenecks and areas of concern in a parallel encryption implementation. The analysis first identifies issues such as suboptimal data chunking, overhead in parallel function execution, lack of accounting for sequential encryption time, and uneven task distribution.
To address these concerns and optimize the performance, the analysis then presents several recommendations. Firstly, it suggests implementing dynamic chunking to determine chunk size based on available CPU resources and input size. Secondly, it proposes using asynchronous processing instead of the pool.map()
function to reduce performance overhead. Furthermore, it emphasizes the importance of measuring sequential encryption time accurately to obtain correct performance measurements. Lastly, it recommends load balancing techniques to distribute workload efficiently among worker processes.
Overall, implementing these recommendations and considering the specific requirements of the encryption operation can significantly enhance the performance of the parallel encryption function.