Code Best Practices Checker | SQL
Refactored PL/SQL Code for Employee Table Iteration
This refactored PL/SQL code eliminates the need for explicit cursor operations and simplifies the iteration over employee names in the employees_table. It improves code readability and conciseness, while also suggesting the use of DBMS_OUTPUT.PUT_LIN...
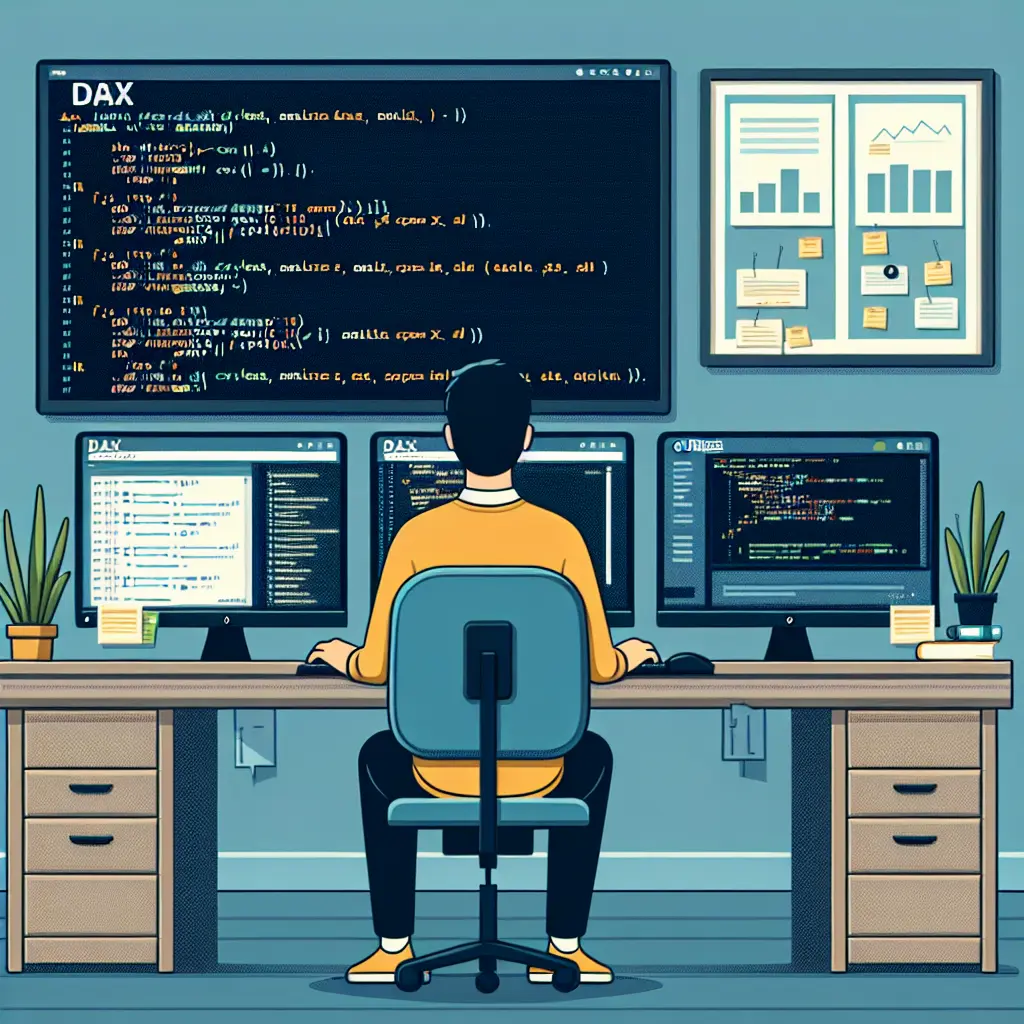
This Query related with thread "SQL Function with Multiple Loops"
Prompt
DECLARE
cursor1 CURSOR FOR SELECT employee_name FROM employees_table;
emp_name VARCHAR2(50);
BEGIN
OPEN cursor1;
LOOP
FETCH cursor1 INTO emp_name;
EXIT WHEN cursor1%NOTFOUND;
-- Perform operations on emp_name
-- e.g. PRINT emp_name;
END LOOP;
CLOSE cursor1;
END;
Answer
Language: PL/SQL
Refactored code:
BEGIN
FOR emp_rec IN (SELECT employee_name FROM employees_table)
LOOP
-- Perform operations on emp_rec.employee_name
-- e.g. DBMS_OUTPUT.PUT_LINE(emp_rec.employee_name);
END LOOP;
END;
Main Changes:
- Removed the
DECLARE
keyword as it is not required in the refactored version. - Replaced the cursor declaration and opening with a simplified FOR loop that iterates over the result set directly.
- Removed the explicit FETCH statement as it is not required in the FOR loop.
- Replaced the
%NOTFOUND
condition with a simplified loop exit condition using the implicit cursor attribute%FOUND
. - Replaced the
PRINT
statement withDBMS_OUTPUT.PUT_LINE
to display the emp_name (commented out in the refactored version). - Removed the explicit CLOSE statement as it is not required in the refactored version.
Reasoning:
- The refactored version eliminates the need for explicit cursor declaration, opening, fetching, and closing, making the code more concise and readable.
- The FOR loop simplifies the iteration over the result set, implicitly handling the cursor operations.
- The use of
DBMS_OUTPUT.PUT_LINE
allows for printing output in PL/SQL.
Additional Suggestions:
- Consider adding exception handling to deal with any potential errors during the iteration process.
- Evaluate if there are any existing indexes or performance considerations that could be applied to optimize the query execution.
Description
More Code Best Practices Checkers
Apache Flink Code Best Practices CheckerApache Pig Code Best Practices CheckerAzure Data Factory Code Best Practices CheckerC/C++ Code Best Practices CheckerCouchDB Code Best Practices CheckerDAX Code Best Practices CheckerExcel Code Best Practices CheckerFirebase Code Best Practices CheckerGoogle BigQuery Code Best Practices CheckerGoogle Sheets Code Best Practices CheckerGraphQL Code Best Practices CheckerHive Code Best Practices CheckerJava Code Best Practices CheckerJavaScript Code Best Practices CheckerJulia Code Best Practices CheckerLua Code Best Practices CheckerM (Power Query) Code Best Practices CheckerMATLAB Code Best Practices CheckerMongoDB Code Best Practices CheckerOracle Code Best Practices CheckerPostgreSQL Code Best Practices CheckerPower BI Code Best Practices CheckerPython Code Best Practices CheckerR Code Best Practices CheckerRedis Code Best Practices CheckerRegex Code Best Practices CheckerRuby Code Best Practices CheckerSAS Code Best Practices CheckerScala Code Best Practices CheckerShell Code Best Practices CheckerSPSS Code Best Practices CheckerSQL Code Best Practices CheckerSQLite Code Best Practices CheckerStata Code Best Practices CheckerTableau Code Best Practices CheckerVBA Code Best Practices Checker