Code Issues Solver | JavaScript
JavaScript Code for Appending Text to HTML Element
This code demonstrates how to select an HTML element by its ID, create a new paragraph element, set the text content of the paragraph, and append it to the selected element. The example provided adds the "Hello, World!" text to the chosen output elem...
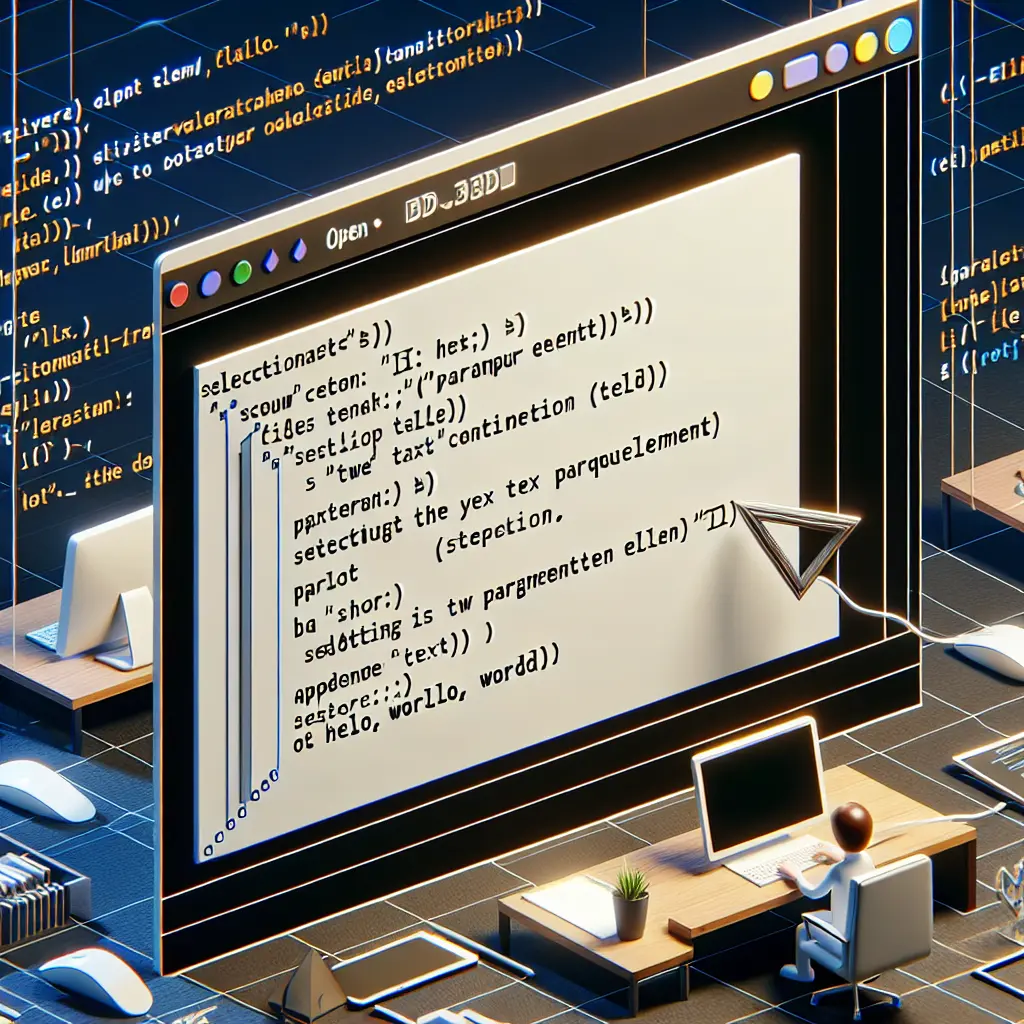
This Query related with thread "Methods to Select HTML Elements by ID in JavaScript"
Prompt
// Select an HTML element by its ID
var outputElement = document.getElementById("output");
// Create a new paragraph element
var paragraph = document.createElement("p");
// Set the text content of the paragraph
paragraph.textContent = "Hello, World!";
// Append the paragraph to the selected element
outputElement.appendChild(paragraph);
Answer
The code provided selects an HTML element by its ID, creates a new paragraph element, sets the text content of the paragraph, and appends the paragraph to the selected element. It effectively adds the "Hello, World!" text to the chosen output element in the HTML document.
// JavaScript solution
// Select an HTML element by its ID
var outputElement = document.getElementById("output");
// Create a new paragraph element
var paragraph = document.createElement("p");
// Set the text content of the paragraph
paragraph.textContent = "Hello, World!";
// Append the paragraph to the selected element
outputElement.appendChild(paragraph);
Example: HTML:
<div id="output"></div>
JavaScript:
// Select an HTML element by its ID
var outputElement = document.getElementById("output");
// Create a new paragraph element
var paragraph = document.createElement("p");
// Set the text content of the paragraph
paragraph.textContent = "Hello, World!";
// Append the paragraph to the selected element
outputElement.appendChild(paragraph);
Unit Testing:
- Test that the output element exists and is selected correctly by its ID.
- Test that a new paragraph element is created.
- Test that the text content of the paragraph is set to "Hello, World!".
- Test that the paragraph is successfully appended to the selected output element.
Description
This code demonstrates how to select an HTML element by its ID, create a new paragraph element, set the text content of the paragraph, and append it to the selected element. The example provided adds the "Hello, World!" text to the chosen output element in the HTML document. Unit testing is also suggested to verify the functionality of the code.