Pseudo Code Generator | JavaScript
Pseudocode for Task Class Analysis
This pseudocode defines a Task class and demonstrates the structure and logic for creating and manipulating tasks. It includes functionality for creating a task, editing a task's priority, and sorting tasks based on priority or due date.
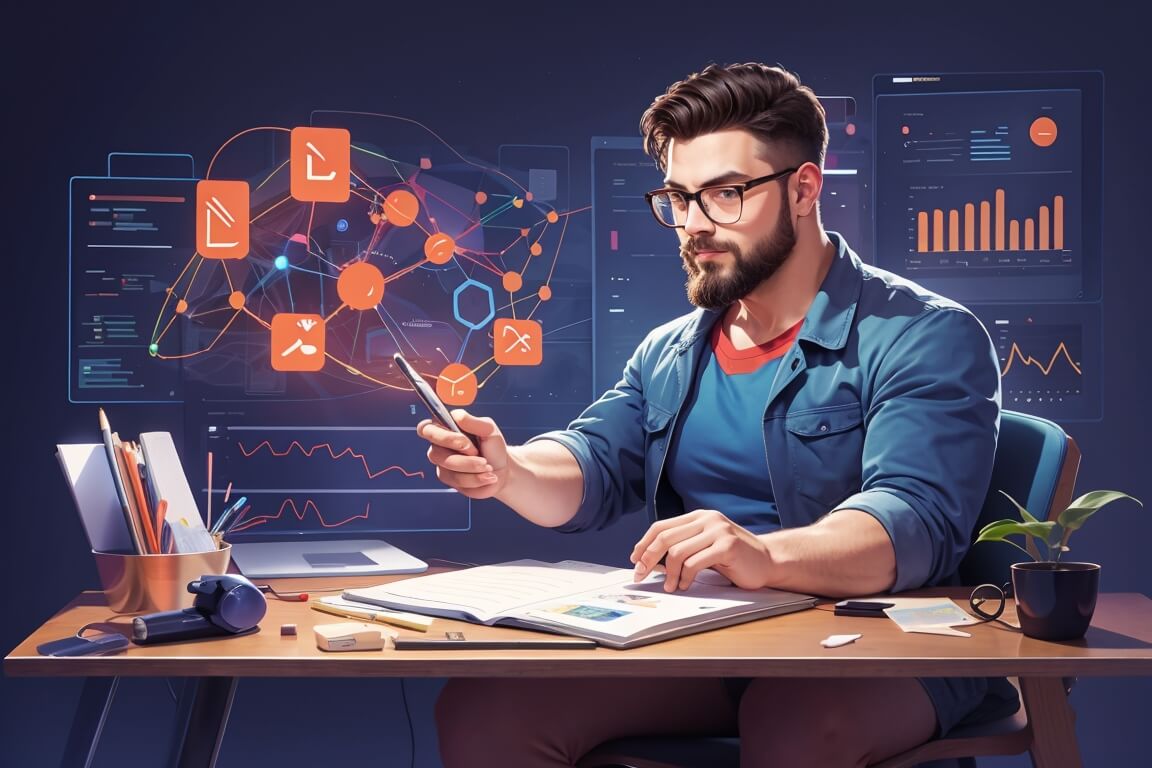
This Query related with thread "Async/Await Example OVERVIEW: Demonstrates how to implement an asynchr..."
Prompt
class Task:
title: string
description: string
due_date: date
priority: int
# Creating a task
task = Task()
task.title = input("Enter task title: ")
task.description = input("Enter task description: ")
task.due_date = input("Enter task due date: ")
task.priority = input("Enter task priority level: ")
# Editing a task's priority
new_priority = input("Enter new priority level: ")
if validate_priority(new_priority):
task.priority = new_priority
# Sorting tasks by priority
if user_input == "sort by priority":
sort_tasks_by_priority(tasks)
elif user_input == "sort by due date":
sort_tasks_by_due_date(tasks)
else:
print("Invalid sorting option")
# Helper function to validate priority level
def validate_priority(priority):
if priority.isdigit() and int(priority) >= 1 and int(priority) <= 5:
return True
else:
print("Invalid priority level")
return False
# Sorting tasks by priority helper function
def sort_tasks_by_priority(tasks):
tasks.sort(key=lambda x: x.priority)
# Sorting tasks by due date helper function
def sort_tasks_by_due_date(tasks):
tasks.sort(key=lambda x: x.due_date)
Answer
Pseudocode for Task Class
# Task class definition
class Task:
title: string
description: string
due_date: date
priority: int
# Creating a task
task = Task()
task.title = input("Enter task title: ")
task.description = input("Enter task description: ")
task.due_date = input("Enter task due date: ")
task.priority = input("Enter task priority level: ")
# Editing a task's priority
new_priority = input("Enter new priority level: ")
if validate_priority(new_priority):
task.priority = new_priority
# Sorting tasks by priority
if user_input == "sort by priority":
sort_tasks_by_priority(tasks)
elif user_input == "sort by due date":
sort_tasks_by_due_date(tasks)
else:
print("Invalid sorting option")
# Helper function to validate priority level
def validate_priority(priority):
if priority is a valid integer between 1 and 5:
return True
else:
print("Invalid priority level")
return False
# Sorting tasks by priority helper function
def sort_tasks_by_priority(tasks):
sort tasks based on priority
# Sorting tasks by due date helper function
def sort_tasks_by_due_date(tasks):
sort tasks based on due date
Notes:
- The pseudocode demonstrates the main structure and logic of the problem.
- It defines a
Task
class with propertiestitle
,description
,due_date
, andpriority
. - It creates a task object (
task
) by taking input from the user for the task's properties. - It allows the user to edit the task's priority by validating and setting a new priority level.
- It provides options to sort tasks by priority or due date based on user input.
- It includes helper functions
validate_priority
,sort_tasks_by_priority
, andsort_tasks_by_due_date
to validate priority levels and sort tasks.
Description
The pseudocode begins by defining a Task
class with properties title
, description
, due_date
, and priority
. It then creates a task object by taking input from the user for the task's properties. The user can also edit the task's priority by entering a new priority level, which is validated before being set. The pseudocode provides options to sort tasks by priority or due date based on user input. It includes helper functions validate_priority
, sort_tasks_by_priority
, and sort_tasks_by_due_date
to handle the validation of priority levels and sorting of tasks. Overall, this pseudocode provides a clear and concise structure for managing tasks in a program.